Chapter 2 Assignment Page
Simple Sequence Control Structure
NOTE: The understanding
of the method the computer uses to solve problems is critical to the rest
of the course. When you solve each of the problems in the chapter use the
memory diagrams and walk through the steps one at a time as if you are
the computer system.
-
Algorithm – a step-by-step procedure to solve a problem. NOTE: Beginning
with this chapter, the you will develop algorithms for the problems in
the chapter using either flowcharting.
-
Characteristics of an algorithm – algorithms must use only operations
for a given set of basic operations and produce the problem solution, or
answer, in a finite number of such operations. NOTE: the algorithm must
only use the three control structures simple sequence, selection and iteration.
This chapter focuses on the simple sequence control structure. Chapter
3 covers the selection control structure. Chapters 4 and 5 cover the iteration
control structure.
-
Character, field, record, and file: NOTE: data is input into the computer
system in the form of a record. The records are stored in files on a secondary
storage device or input by the user. Cover the terms character, field,
record and file and then start solving problems listed at the end of the
chapter. Gradually add in the other concepts for the chapter as they are
needed.
Character – A character is a letter, number or special character.
A character is any key pressed on the keyboard.
Field - a single piece of data, or fact, about a single entity in
a file. For example, an employee number might be one of the fields in a
payroll record. A field is made up of characters.
Record – a collection of data, or facts about a single entity in
the file. A record is made up of fields.
File - a file is a collection of related data or facts. A file is
made up of records.
-
Function of Random Access Memory in reference to the memory diagrams
used in the chapter – when data is input into the computer system it is
initially stored in Random Access Memory. If the data is not stored in
Random Access Memory, the computer system can not access the data. NOTE:
the problems solved in this chapter illustrate the use of memory diagrams.
The memory diagrams will show you what data is stored in memory.
-
Variables, constants and assignment statements - a memory location must
be created to store data in memory. The data can either be stored as a
variable or a constant.
Variable - A variable stores data items whose values may change,
or vary, during processing. Variable names are created to represent these
data items. Examples of variables are name, address and social security
number. When data is input into the computer system, you will not have
an entire file with all names, addresses and social security numbers the
same. Therefore, the data is stored in a variable name so that the data
values can change.
Example: Read Name, Address, SSN - This statement will cause the
computer system to transfer data from an input file into RAM. The data
will be stored in the three memory locations created called name, address
and SSN.
Constant - a constant is a value that never changes. You can either
use the constant directly in the program design or create a variable name
to store the constant. An example of a constant is the sales tax. If you
are designing a program that uses the sales tax, the amount will not change
from one input record to the next.
Example: Sales Tax = 4.5%
Assignment - if additional data is need to solve a program, an assignment
statement must be created. An assignment statement must adhere to the following
rules of formation:
-
Only a single variable name may appear to the left of the assignment
symbol, which in our example is =.
-
Only a single variable name, constant, or expression may appear to the
right of the =.
-
Everything to the right of the = must be known (defined) to the computer.
Example: Sale = Price * Sales Tax
-
Mathematical order of operations – when you are creating assignment
statements, you need to use the mathematical order of operations. Computer
systems use the same order of operations to solve problems. The math operations
are solved in this order. PEMDAS – Parenthesis, Exponents, Multiplication,
Division, Addition and Subtraction. Multiplication and Division are on
the same level. Addition and Subtraction are on the same level. When the
computer deals with operators on the same level, they are processed from
left to right. NOTE: Problem #9 on page 34 reviews the order of operations.
Example: (2 + 3) * 6 =
5 * 6 = 30
-
The statement in the parenthesis is performed first.
-
Multiplication is performed last.
The same formula without the parenthesis will give a different
result.
2 + 3 * 6 =
2 + 18 = 20
-
Multiplication is performed first.
-
Addition is performed last.
-
General I/O, process, flowlines, and terminal interrupt symbols – NOTE:
Use your flowcharting template or flip to Appendix A on page 309 in the
Tools for Structured Design textbook to identify the symbols. This section
covers the four basic flowcharting symbols used in this chapter. The other
symbols will be introduced in future chapters.
Input/Output Symbol – this symbol is used to input data into the
computer system and output information from the computer system. When the
symbol is used for input, the word READ is placed in the symbol with the
variable locations where the data will be stored. When the symbol is used
for output, the word WRITE is placed in the symbol with the variable locations
that need to be output. The information is output in the order it appears
on the WRITE statement.
Process Symbol – used for any processing step, an operation or group
of operations causing change in value, form or location of data. This symbol
is used for all assignment statements.
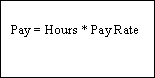
Flowline Symbol – represent sequence of operations and direction
of data flow. Arrowheads are required if linkage is not top-to-bottom or
left-to-right. If no arrowheads are list, the direction of data flow is
assumed
to be top-to-bottom or left-to-right.
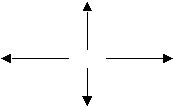
Terminal Interrupt Symbol – this marks the terminal point in a flowchart.
It is placed at the beginning and end of each flowchart or flowchart segment.
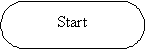
Exercises: In Chapter 2, the lab problems allow you to trace through
flowchart designs in the textbook. You will also create your own flowcharts.
It is important to be methodical when you cover the solutions to the problems.
Although you can not memorize the solution to each problem in the book,
you can learn the method to solving the problems. You should first list
the input that is given in the problem, second list the output that is
needed, compare the output that is needed to the input that is given, if
they match then they should cross it off the output list. Any of the variables
that are left on the output list require processing statements. Before
the solution to the problem is solved, the processing steps need to be
placed in the correct order.
Problem #9 - These problems practice the mathematical order of operations.
Problem #10 – With this problem, you will take input data and walk
it through a flowchart. Draw an empty box to represent memory. Walk through
each step of the flowchart one step at a time, recognize the use of the
flowcharting symbol, create the locations for the variables in memory and
place the data in the locations. Using this problem, the you will understand
how the computer system processes data. The problem uses many mathematical
operations. The problem using many mathematical operations. You may need
a calculator to solve the mathematical operations.
Problem #11 – This problem is the same as #10. Define the terms variable
and constant. Step d requires you to walk through the flowchart using the
given input and memory diagrams.
Problem #13 – This is the first full problem the you will design.
You need to create variable names. The variable names should be descriptive,
but kept to one or two words. Place three columns on a sheet of paper labeled
input, output and processing. List the input given and output required.
Compare the input and output. The variables left in the output column require
processing. At this point, there is no major difference between the flowchart
and pseudocode solution.
Problem #15 – In this problem, you need to create five separate locations
for each grade to be stored. You also need to use parenthesis to calculate
the total first and then divide the total to calculate the average.
Problems #16 & #17 - These problems are difficult since most
people do not remember the formulas for perimeter, area and circumference.
Once you have completed the input, output and processing columns, if you
do not remember the formulas, leave that portion blank. Normally these
types of formulas they can look up in a book. You will not need to know
these formulas anywhere else in the class.